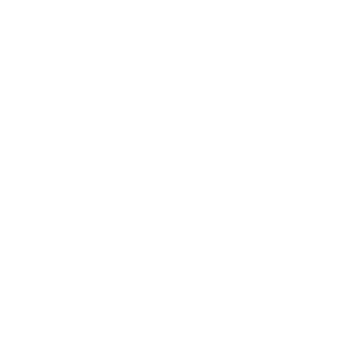
Description
Roman numerals are represented by seven different symbols: , , , , , and .IVXLCDM
Symbol Value
I 1
V 5
X 10
L 50
C 100
D 500
M 1000
For example, is written as in Roman numeral, just two ones added together. is written as , which is simply . The number is written as , which is .2II12XIIX + II27XXVIIXX + V + II
Roman numerals are usually written largest to smallest from left to right. However, the numeral for four is not . Instead, the number four is written as . Because the one is before the five we subtract it making four. The same principle applies to the number nine, which is written as . There are six instances where subtraction is used:IIIIIVIX
I can be placed before (5) and (10) to make 4 and 9. VX
X can be placed before (50) and (100) to make 40 and 90. LC
C can be placed before (500) and (1000) to make 400 and 900.DM
Given a roman numeral, convert it to an integer.
Example 1:
Input: s = "III"
Output: 3
Explanation: III = 3.
Example 2:
Input: s = "LVIII"
Output: 58
Explanation: L = 50, V= 5, III = 3.
Example 3:
Input: s = "MCMXCIV"
Output: 1994
Explanation: M = 1000, CM = 900, XC = 90 and IV = 4.
Constraints:
1 <= s.length <= 15
s contains only the characters .('I', 'V', 'X', 'L', 'C', 'D', 'M')
It is guaranteed that is a valid roman numeral in the range .s[1, 3999]
Code
package main
import "fmt"
func main() {
var inp string
fmt.Scan(&inp)
fmt.Println(romanToInt(inp))
}
func romanToInt(s string) int {
romanMap := map[byte]int{
'I': 1,
'V': 5,
'X': 10,
'L': 50,
'C': 100,
'D': 500,
'M': 1000,
}
output := 0
for i := 0; i < len(s); i++ {
placed := false
if i + 1 < len(s) {
// I가 I + 1 보다 작다면 ...
if romanMap[s[i + 1]] > romanMap[s[i]] {
output += romanMap[s[i + 1]] - romanMap[s[i]]
placed = true
i++
}
}
if !placed {
output += romanMap[s[i]]
}
}
return output
}
시간 복잡도는 여전히 O(n)으로, 문자열의 길이에 비례하여 효율적으로 동작합니다.
'leetcode' 카테고리의 다른 글
20. Valid Parentheses (0) | 2024.07.12 |
---|---|
14. Longest Common Prefix (0) | 2024.07.12 |
9. Palindrome Number (0) | 2024.05.17 |
908. Smallest Range I (0) | 2024.05.16 |
2582. Pass the Pillow (0) | 2024.05.16 |