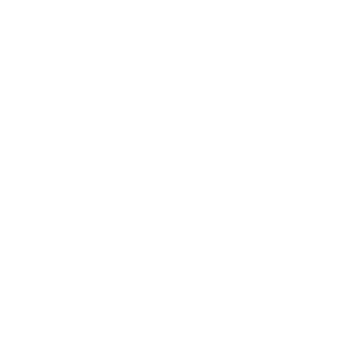
Description
Easy
Topics
Companies
Write a function to find the longest common prefix string amongst an array of strings.
If there is no common prefix, return an empty string "".
Example 1:
Input: strs = ["flower","flow","flight"]
Output: "fl"
Example 2:
Input: strs = ["dog","racecar","car"]
Output: ""
Explanation: There is no common prefix among the input strings.
Constraints:
1 <= strs.length <= 200
0 <= strs[i].length <= 200
strs[i] consists of only lowercase English letters.
Code
package main
import (
"fmt"
)
func main() {
fmt.Println( longestCommonPrefix([]string{"flower","flow","flight"}) )
}
func longestCommonPrefix(strs []string) string {
fix := strs[0]
for i := range len(fix) {
for _, word := range strs[1:] {
if i == len(word) || fix[i] != word[i] {
return fix[0:i]
}
}
}
return fix
}
이 함수의 시간 복잡도는 O(m * n)입니다. m은 첫 번째 문자열의 길이이고, n은 문자열 배열의 크기입니다.
'leetcode' 카테고리의 다른 글
21. Merge Two Sorted Lists (0) | 2024.07.17 |
---|---|
20. Valid Parentheses (0) | 2024.07.12 |
13. Roman to Integer (0) | 2024.07.02 |
9. Palindrome Number (0) | 2024.05.17 |
908. Smallest Range I (0) | 2024.05.16 |