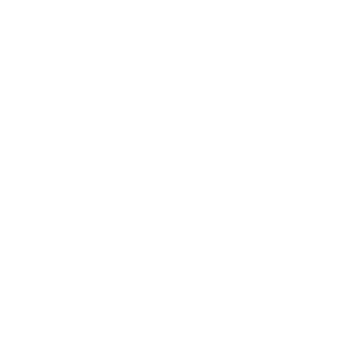
Description
20. Valid Parentheses
Easy
Topics
Companies
Hint
Given a string s containing just the characters '(', ')', '{', '}', '[' and ']', determine if the input string is valid.
An input string is valid if:
Open brackets must be closed by the same type of brackets.
Open brackets must be closed in the correct order.
Every close bracket has a corresponding open bracket of the same type.
Example 1:
Input: s = "()"
Output: true
Example 2:
Input: s = "()[]{}"
Output: true
Example 3:
Input: s = "(]"
Output: false
Constraints:
1 <= s.length <= 104
s consists of parentheses only '()[]{}'.
Code
package main
import "fmt"
func main() {
fmt.Println( isValid("[([])]") )
}
type Stack struct {
data []string
}
func (s *Stack) push(v string) {
s.data = append(s.data, v)
}
func (s *Stack) pop() string {
if len(s.data) == 0 {
return ""
}
v := s.data[len(s.data)-1]
s.data = s.data[:len(s.data)-1]
return v
}
func isValid(s string) bool {
if s == "" {
return true
}
stack := Stack{}
for _, v := range s {
if v == '(' || v == '[' || v == '{' {
stack.push(string(v))
} else {
if len(stack.data) == 0 {
return false
}
top := stack.pop()
if (v == ')' && top != "(") || (v == ']' && top != "[") || (v == '}' && top != "{") {
return false
}
}
}
return true
}
- 문자열 s의 모든 문자를 한 번씩 순회합니다.
- 문자열의 길이를 n이라고 하면, 주 반복문은 O(n)입니다.
'leetcode' 카테고리의 다른 글
27. Remove Element (0) | 2024.08.06 |
---|---|
21. Merge Two Sorted Lists (0) | 2024.07.17 |
14. Longest Common Prefix (0) | 2024.07.12 |
13. Roman to Integer (0) | 2024.07.02 |
9. Palindrome Number (0) | 2024.05.17 |