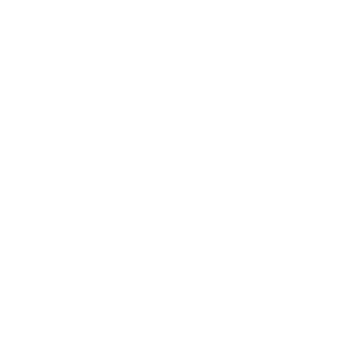
Description
There are n people standing in a line labeled from 1 to n. The first person in the line is holding a pillow initially. Every second, the person holding the pillow passes it to the next person standing in the line. Once the pillow reaches the end of the line, the direction changes, and people continue passing the pillow in the opposite direction.
For example, once the pillow reaches the nth person they pass it to the n - 1th person, then to the n - 2th person and so on.
Given the two positive integers n and time, return the index of the person holding the pillow after time seconds.
Example 1:
Input: n = 4, time = 5
Output: 2
Explanation: People pass the pillow in the following way: 1 -> 2 -> 3 -> 4 -> 3 -> 2.
Afer five seconds, the pillow is given to the 2nd person.
Example 2:
Input: n = 3, time = 2
Output: 3
Explanation: People pass the pillow in the following way: 1 -> 2 -> 3.
Afer two seconds, the pillow is given to the 3rd person.
Constraints:
2 <= n <= 1000
1 <= time <= 1000
Code
func passThePillow(n int, time int) int {
output := 1
cal_type := 1
time_check := 0
for i := 1; time_check != time; i++ {
if i%n == 0 {
cal_type *= -1
output += cal_type
i = 1
} else {
output += cal_type
}
time_check++
}
return output
}
passThePillow 함수는 주어진 시간 동안 어느 사람이 베개를 가지고 있는지를 결정하는 함수입니다. 매개변수 n은 사람의 수이고, time은 지난 시간입니다. 각 사람은 순차적으로 베개를 가지고 있으며, 시간이 경과함에 따라 베개를 넘겨주는 패턴을 따릅니다.
이 코드는 반복문을 사용하여 각 시간마다 베개를 가지고 있는 사람을 계산합니다. cal_type 변수는 베개를 가지고 있는 사람이 변경될 때 사용되며, time_check 변수는 현재까지 지난 시간을 나타냅니다.
내부 반복문에서 i를 n으로 나누었을 때 나머지가 0인 경우, 다음 사람으로 베개를 넘겨야 하므로 cal_type를 변경하고 다음 사람으로 넘어갑니다. 그렇지 않은 경우에는 현재 사람이 베개를 가지고 있으므로 output에 cal_type을 더해줍니다.
이 코드의 시간 복잡도는 O(time)입니다. 외부 반복문은 주어진 시간만큼 반복되며, 내부 반복문은 상수 시간 내에 실행됩니다. 따라서 전체적으로는 입력된 시간에 비례하는 선형 시간이 소요됩니다.
'leetcode' 카테고리의 다른 글
9. Palindrome Number (0) | 2024.05.17 |
---|---|
908. Smallest Range I (0) | 2024.05.16 |
506. Relative Ranks (0) | 2024.05.16 |
383. Ransom Note (0) | 2024.05.16 |
876. Middle of the Linked List (0) | 2024.05.02 |