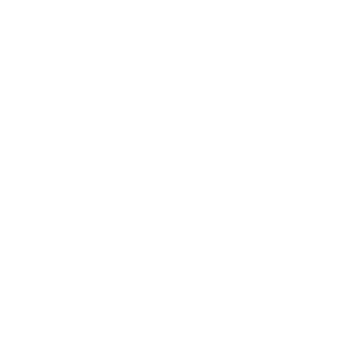
Description
Given an integer , return if is a xtruex
palindrome
, and otherwise.false
Example 1:
Input: x = 121
Output: true
Explanation: 121 reads as 121 from left to right and from right to left.
Example 2:
Input: x = -121
Output: false
Explanation: From left to right, it reads -121. From right to left, it becomes 121-. Therefore it is not a palindrome.
Example 3:
Input: x = 10
Output: false
Explanation: Reads 01 from right to left. Therefore it is not a palindrome.
Constraints:
-231 <= x <= 231 - 1
Follow up: Could you solve it without converting the integer to a string?
Code
func isPalindrome(x int) bool {
var ans bool
if x < 0 {
return ans
}
nums := make([]int, 0)
for {
division := x / 10
if division == 0 {
nums = append(nums, x)
break
}
lastDigit := x - division*10
x = division
nums = append(nums, lastDigit)
}
left, right := 0, len(nums)-1
for {
if left >= right {
ans = true
break
}
if nums[left] != nums[right] {
break
}
left += 1
right -= 1
}
return ans
}
시간 복잡도는 O(log10(n))입니다. 숫자의 자리수를 절반만큼만 처리하기 때문
'leetcode' 카테고리의 다른 글
14. Longest Common Prefix (0) | 2024.07.12 |
---|---|
13. Roman to Integer (0) | 2024.07.02 |
908. Smallest Range I (0) | 2024.05.16 |
2582. Pass the Pillow (0) | 2024.05.16 |
506. Relative Ranks (0) | 2024.05.16 |