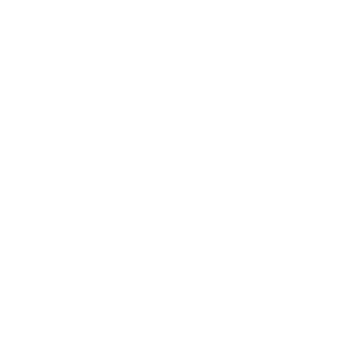
Description
You are given an integer array score of size n, where score[i] is the score of the ith athlete in a competition. All the scores are guaranteed to be unique.
The athletes are placed based on their scores, where the 1st place athlete has the highest score, the 2nd place athlete has the 2nd highest score, and so on. The placement of each athlete determines their rank:
The 1st place athlete's rank is "Gold Medal".
The 2nd place athlete's rank is "Silver Medal".
The 3rd place athlete's rank is "Bronze Medal".
For the 4th place to the nth place athlete, their rank is their placement number (i.e., the xth place athlete's rank is "x").
Return an array answer of size n where answer[i] is the rank of the ith athlete.
Example 1:
Input: score = [5,4,3,2,1]
Output: ["Gold Medal","Silver Medal","Bronze Medal","4","5"]
Explanation: The placements are [1st, 2nd, 3rd, 4th, 5th].
Example 2:
Input: score = [10,3,8,9,4]
Output: ["Gold Medal","5","Bronze Medal","Silver Medal","4"]
Explanation: The placements are [1st, 5th, 3rd, 2nd, 4th].
Constraints:
n == score.length
1 <= n <= 104
0 <= score[i] <= 106
All the values in score are unique.
Code
func findRelativeRanks(score []int) []string {
output := make([]string, len(score))
for index_st, value_st := range score {
rank := 1
for _, value_nd := range score {
if value_st < value_nd {
rank++
}
}
switch rank {
case 1:
output[index_st] = "Gold Medal"
case 2:
output[index_st] = "Silver Medal"
case 3:
output[index_st] = "Bronze Medal"
default:
output[index_st] = strconv.Itoa(rank)
}
}
return output
}
findRelativeRanks는 주어진 점수 배열에 대해 각 점수의 순위를 문자열로 반환합니다. 순위는 점수가 높을수록 높은 순위를 가지며, 동일한 점수의 경우 동일한 순위를 부여합니다.
이 함수는 각 점수를 순회하면서 해당 점수보다 큰 점수의 개수를 세어 순위를 결정합니다. 그리고 순위에 따라 점수를 문자열로 변환하여 출력합니다.
이 코드의 시간 복잡도는 O(n^2)입니다. 외부 반복문은 점수 배열을 한 번 순회하며, 내부 반복문은 각 점수마다 전체 점수 배열을 순회하므로 이중 반복문이 실행됩니다. 따라서 전체적으로는 O(n^2)의 시간이 소요됩니다. 이러한 반복문 중첩은 대규모 데이터셋에서는 비효율적일 수 있습니다.
'leetcode , 백준' 카테고리의 다른 글
908. Smallest Range I (1) | 2024.05.16 |
---|---|
2582. Pass the Pillow (0) | 2024.05.16 |
383. Ransom Note (0) | 2024.05.16 |
876. Middle of the Linked List (0) | 2024.05.02 |
1342. Number of Steps to Reduce a Number to Zero (0) | 2024.05.02 |