마이그레이션 이유
TypeORM은 TypeScript와 완벽하게 호환되어, TypeScript의 장점을 온전히 누릴 수 있다고 공식 문서에 적혀있다...
개발자가 데이터베이스와의 연결을 관리하는 데 필요한 노력을 줄여주는데 SQL 쿼리를 작성하는 대신, TypeORM의 메서드를 사용하면 데이터베이스를 조작할 수 있다.
또한, TypeORM은 자동으로 SQL을 생성하므로, 복잡한 쿼리 작성의 부담을 덜어주며, 개발 속도를 크게 향상 시켜주기 때문에 TypeORM을 적용하려고 하고 있다.
TypeORM을 기존 코드에 적용하려면 데이터베이스 연결 설정 및 엔티티 정의를 추가해야 합니다.
# 패키지 설치
npm install typeorm reflect-metadata pg
TypeORM 설정
TypeORM의 데이터 소스를 설정하기 위한 파일, 기존의 pg.Pool 설정을 대체하여 작성
import 'reflect-metadata'; // TypeORM이 필요로 함
import { DataSource } from 'typeorm';
import dotenv from 'dotenv';
dotenv.config();
// 데이터 소스 설정
export const AppDataSource = new DataSource({
type: 'postgres',
host: process.env.DB_HOST,
port: parseInt(process.env.DB_PORT || '5432', 10),
username: process.env.DB_USER,
password: process.env.DB_PASSWORD,
database: process.env.DB_NAME,
synchronize: true, // 개발 환경에서만 true (자동 마이그레이션)
logging: true, // 쿼리 로그 활성화
entities: ['./src/entities/*.ts'], // 엔티티 경로
});
데이터베이스 초기화
애플리케이션이 시작되기 전에 데이터베이스 연결을 초기화
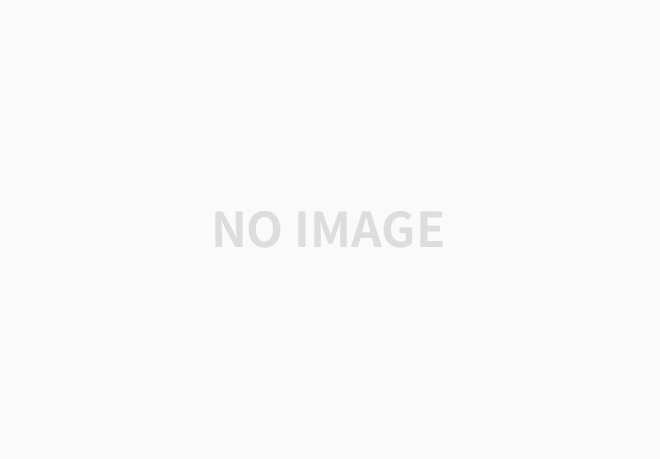
최상단 서버를 실행하는 파일에서 import를 무조건 해줘야 함 ( 동작이 안 됨 )
import 'reflect-metadata'; // Required for TypeORM
Entity 생성 ( 모델 )
import { Entity, PrimaryGeneratedColumn, Column, CreateDateColumn, UpdateDateColumn } from 'typeorm';
@Entity('users') // 데이터베이스 테이블 이름
export class User {
@PrimaryGeneratedColumn()
id!: number; // 기본 키
@Column({ type: 'varchar', length: 100 })
name!: string; // 사용자 이름
@Column({ type: 'varchar', length: 200, unique: true })
email!: string; // 이메일 (유니크)
@Column({ type: 'varchar', length: 255 })
password!: string; // 비밀번호
@Column({ type: 'varchar', length: 255, nullable: true })
avatar?: string; // 프로필 이미지 (nullable)
@CreateDateColumn()
createdAt!: Date; // 생성일자 (자동 생성)
@UpdateDateColumn()
updatedAt!: Date; // 수정일자 (자동 업데이트)
}
컴파일 에러가 뜬다면 ?
# tsConfig.ts 에 주석을 풀어 true로 변경
"experimentalDecorators": true, /* Enable experimental support for legacy experimental decorators. */
"emitDecoratorMetadata": true, /* Emit design-type metadata for decorated declarations in source files. */
결과
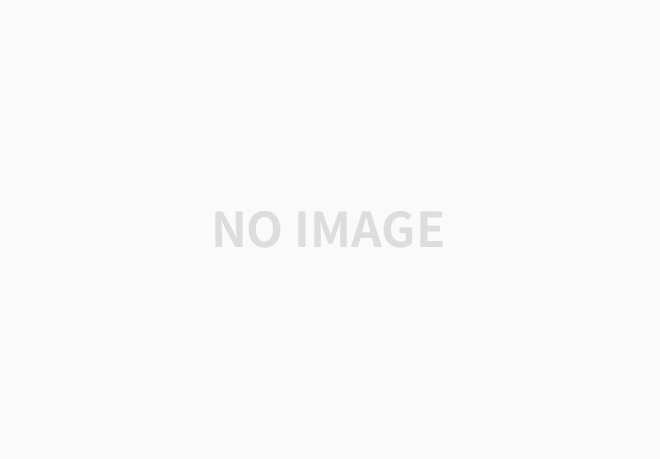
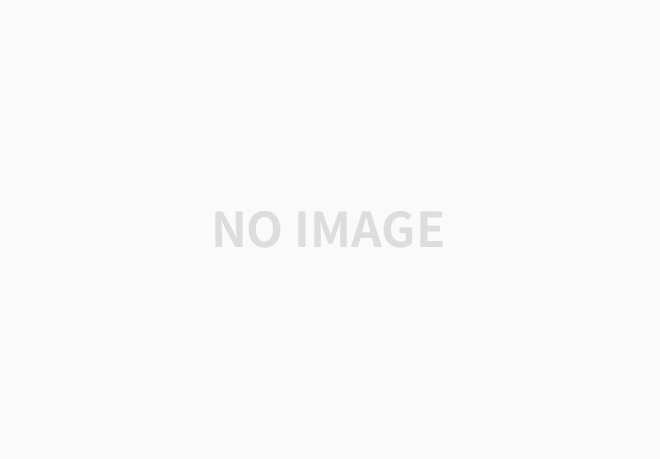
서버 실행 시에 작성한 엔티티에 맞게 테이블이 생기며 엔티티 코드 변경 시에 자동으로 테이블이 마이그레이션 되는 것도 확인.
'Nodejs' 카테고리의 다른 글
NodeJS 로그인 기능 구현 (0) | 2024.12.20 |
---|---|
NodeJS 회원가입 기능 구현 (2) | 2024.12.20 |
Nodejs PostgreSQL 연동 (2) | 2024.12.18 |
JWT, Bcrypt 예제 (0) | 2024.12.18 |
Nodejs 개발환경 셋팅 (0) | 2024.12.18 |