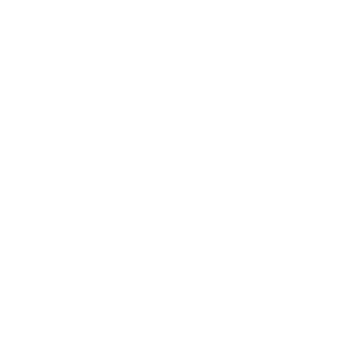
Description
Easy
Topics
Companies
Given a string s consisting of words and spaces, return the length of the last word in the string.
A word is a maximal
substring
consisting of non-space characters only.
Example 1:
Input: s = "Hello World"
Output: 5
Explanation: The last word is "World" with length 5.
Example 2:
Input: s = " fly me to the moon "
Output: 4
Explanation: The last word is "moon" with length 4.
Example 3:
Input: s = "luffy is still joyboy"
Output: 6
Explanation: The last word is "joyboy" with length 6.
Constraints:
1 <= s.length <= 104
s consists of only English letters and spaces ' '.
There will be at least one word in s.
Code
package main
import (
"fmt"
"strings"
)
func main() {
fmt.Println(lengthOfLastWord("Hello World") == 5)
}
func lengthOfLastWord(s string) int {
words := strings.Fields(s)
return len(words[len(words) - 1])
}
시간 복잡도 분석
- strings.Fields(s):
- 이 함수는 입력 문자열 s를 공백으로 분할하여 단어들의 슬라이스를 생성합니다.
- 이 연산의 시간 복잡도는 O(n)입니다. 여기서 n은 문자열 s의 길이입니다. 문자열 전체를 한 번 순회하면서 공백을 기준으로 단어를 분리합니다.
- 마지막 단어의 길이 계산:
- len(words[len(words) - 1])은 마지막 단어의 길이를 반환합니다.
- 이 연산은 단어 길이 m에 비례하며, 따라서 O(m)입니다. 여기서 m은 마지막 단어의 길이입니다.
전체 시간 복잡도
- 전체 시간 복잡도는 O(n + m)이 됩니다.
- n은 입력 문자열 s의 길이이며, 이는 문자열을 공백으로 나누는 데 걸리는 시간입니다.
- m은 마지막 단어의 길이로, 이 값을 계산하는 데 소요되는 시간입니다.
- 대부분의 경우 m은 n에 비해 매우 작으므로, 일반적으로 이 함수의 시간 복잡도는 O(n)이라고 할 수 있습니다.
'leetcode , 백준' 카테고리의 다른 글
70. Climbing Stairs (2) | 2024.10.19 |
---|---|
69. Sqrt(x) (1) | 2024.10.19 |
28. Find the Index of the First Occurrence in a String (0) | 2024.08.06 |
35. Search Insert Position (0) | 2024.08.06 |
27. Remove Element (0) | 2024.08.06 |