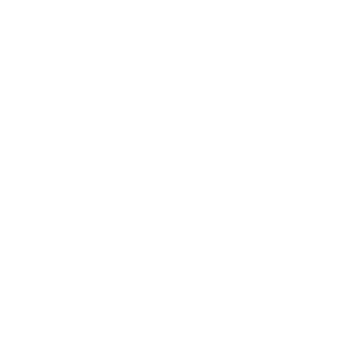
Description
Given an integer n, return a string array answer (1-indexed) where:
answer[i] == "FizzBuzz" if i is divisible by 3 and 5.
answer[i] == "Fizz" if i is divisible by 3.
answer[i] == "Buzz" if i is divisible by 5.
answer[i] == i (as a string) if none of the above conditions are true.
Example 1:
Input: n = 3
Output: ["1","2","Fizz"]
Example 2:
Input: n = 5
Output: ["1","2","Fizz","4","Buzz"]
Example 3:
Input: n = 15
Output: ["1","2","Fizz","4","Buzz","Fizz","7","8","Fizz","Buzz","11","Fizz","13","14","FizzBuzz"]
Constraints:
1 <= n <= 104
Code
// 412. Fizz Buzz
func fizzBuzz(n int) []string {
output := make([]string, n)
for i := 1; i <= n; i++ {
if i%3 == 0 && i%5 == 0 {
output[i-1] = "FizzBuzz"
} else if i%3 == 0 {
output[i-1] = "Fizz"
} else if i%5 == 0 {
output[i-1] = "Buzz"
} else {
output[i-1] = strconv.Itoa(i)
}
}
return output
}
이 함수는 n에 대해 반복문을 실행하고, 각 숫자에 대해 조건을 체크하여 문자열을 배열에 할당합니다. 따라서 주어진 함수의 시간 복잡도는 O(n)입니다. 입력값 n에 비례하는 반복문이 실행되므로 전체적으로 선형 시간이 소요됩니다.
'leetcode , 백준' 카테고리의 다른 글
876. Middle of the Linked List (0) | 2024.05.02 |
---|---|
1342. Number of Steps to Reduce a Number to Zero (0) | 2024.05.02 |
1672. Richest Customer Wealth (0) | 2024.04.29 |
1480. Running Sum of 1d Array (0) | 2024.04.28 |
1. Two Sum (0) | 2024.04.28 |